Simple HTTP API
bsms2.etsol.pk/web_distributor/api/sms.php?username=&password=***&sender=BrandName&mobile=&message=TestSMS
<?php $username = "923*****";///Your Username $password = "*****";///Your Password $mobile = "923001234567";///Recepient Mobile Number $sender = "SenderID"; $message = "Test SMS From sendpk.com"; ////sending sms $post = "sender=".urlencode($sender)."&mobile=".urlencode($mobile)."&message=".urlencode($message).""; $url = "bsms2.etsol.pk/web_distributor/api/sms.php?username=".$username."&password=".$password.""; $ch = curl_init(); $timeout = 10; // set to zero for no timeout curl_setopt($ch, CURLOPT_USERAGENT, 'Mozilla/4.0 (compatible; MSIE 6.0; Windows NT 5.1; SV1)'); curl_setopt($ch, CURLOPT_URL,$url); curl_setopt($ch, CURLOPT_POST, 1); curl_setopt($ch, CURLOPT_POSTFIELDS,$post); curl_setopt($ch, CURLOPT_RETURNTRANSFER, true); curl_setopt ($ch, CURLOPT_CONNECTTIMEOUT, $timeout); $result = curl_exec($ch); /*Print Responce*/ echo $result; ?>
Parameter For PHP API
username | Your Login Username (Required) |
---|---|
password | Your Login Password (Required) |
sender | Your company Name/Brand Name like "xyz" Displayed of Recipient Mobile (Required) |
mobile | Recipient Mobile Number (Required) |
message | Message For Recipient (Required) |
type | Type = unicode for unicode SMS (OPTIONAL) |
date | dd-mm-YYYY Example: 25-02-2014 (OPTIONAL) |
time | hh:mm:ss Example: 21:30:55 (OPTIONAL) |
format | Type is xml and json. Not Case sensitive. If set, the result will be returned according to the type specified. (OPTIONAL) |
Return Response and their Meanings
OK | The message was successfully accepted for delivery. |
---|---|
1 | Invalid Username Or Password |
2 | Please Type Username |
3 | Please Type Password |
4 | Please Type Sender ID |
-4 | Invalid Sender ID This Sender ID Is Not Register From P.T.A OR Sender ID Up to 11 Words Please Type Short Sender ID |
5 | Please Type Receiver Mobile Number |
6 | Please Type Your Message |
-6 | Message Length Exceeded More Than 612 Character (type = Text) OR Message Length Exceeded More Than 338 Character (type = unicode) |
8 | Low Credit Please Recharge Your Credit |
9 | SMS Rejected |
7 | Invalid Recipient Mobile Numbers Please Type Correct Mobile Number |
10 | Error! SMS Sending Failed Please Try Again Or Contact To Support |
11 | Invalid Date For Scheduled! |
Send Single SMS Via HTTP API
Below Simple HTTP GET / POST METHOD example is given.
bsms2.etsol.pk/web_distributor/api/sms.php?username=&password=PASSWORD&sender=BrandName&mobile=&message=test+sms.
Successful Out Put Response
{"success":"true","type":"API","totalprice":"1","totalgsm":"1","remaincredit":"87 SMS","results":[{"status":"OK","messageid":"f41ff6a4-58af-4de0-832a-23ddef3fb325","gsm":"923001234567"}]}
Send Multiple SMS Via HTTP API
Below simple HTTP GET / POST METHOD example is given.
bsms2.etsol.pk/web_distributor/api/sms.php?username=&password=PASSWORD&sender=BrandName&mobile=,923001234567,923331234567&message=test+sms.
Successful Out Put Response
{"success":"true","type":"BULKSMS API","totalprice":"3","totalgsm":"3","remaincredit":"67 SMS","results":[{"status":"OK","messageid":"803bc8ec-2524-422b-a28a-5f2f51c3c454","gsm":"923101234567"},{"status":"OK","messageid":"e6458553-d12b-4ffb-8e73-f1345ac3aa46","gsm":"923001234567"},{"status":"OK","messageid":"fd1be2b5-ff9a-419b-890c-9821e715de36","gsm":"923331234567"}]}
Send Single Unicode SMS Via HTTP API
Below simple HTTP GET / POST METHOD example is given. Please click on it to check api interference
bsms2.etsol.pk/web_distributor/api/sms.php?username=&password=PASSWORD&sender=BrandName&mobile=&message=test+sms&type=unicode
Successful Out Put Response
{"success":"true","type":"Unicode API","totalprice":"1","totalgsm":"1","remaincredit":"87 SMS","results":[{"status":"OK","messageid":"f41ff6a4-58af-4de0-832a-23ddef3fb325","gsm":"923001234567"}]}
Send Multiple Unicode SMS Via HTTP API
Below simple HTTP GET / POST METHOD example is given. Please click on it to check api interference
bsms2.etsol.pk/web_distributor/api/sms.php?username=&password=PASSWORD&sender=BrandName&mobile=,923001234567,923331234567&message=test+sms&type=unicode
Successful Out Put Response
{"success":"true","type":"Unicode BULKSMS API","totalprice":"3","totalgsm":"3","remaincredit":"67 SMS","results":[{"status":"OK","messageid":"803bc8ec-2524-422b-a28a-5f2f51c3c454","gsm":"923101234567"},{"status":"OK","messageid":"e6458553-d12b-4ffb-8e73-f1345ac3aa46","gsm":"923001234567"},{"status":"OK","messageid":"fd1be2b5-ff9a-419b-890c-9821e715de36","gsm":"923331234567"}]}
Send Scheduled SMS for Future Reservation Via JSON API
Below simple HTTP GET / POST METHOD example is given.
bsms2.etsol.pk/web_distributor/api/sms.php?username=&password=PASSWORD&sender=BrandName&mobile=92313,92333,92345&date=12-10-2018&time=15:30:00&message=this+is+schedule+sms+api.
Successful Out Put Response
{"success":"true","type":"Schedule API","totalprice":"5","totalgsm":"1","remaincredit":"57 SMS","results":[{"status":"OK","messageid":"01ffd596-6b2f-4906-8686-6702f4bc67e6","gsm":"923101234567"}]}
.NET API Code
using System; using System.Net; using System.Web; public class Program { public static void Main() { string MyUsername = "userxxx"; //Your Username string MyPassword = "92***"; //Your Password string toNumber = "923****"; //Recepient cell phone number with country code string Masking = "SMS Alert"; //Your Company Brand Name string MessageText = "SMS Sent using .Net"; string jsonResponse = SendSMS(Masking, toNumber, MessageText, MyUsername , MyPassword); Console.Write(jsonResponse); //Console.Read(); //to keep console window open if trying in visual studio } public static string SendSMS(string Masking, string toNumber, string MessageText, string MyUsername , string MyPassword) { String URI = "bsms2.etsol.pk" + "/web_distributor/api/sms.php?" + "username=" + MyUsername + "&password=" + MyPassword + "&sender=" + Masking + "&mobile=" + toNumber + "&message=" + Uri.UnescapeDataString(MessageText); // Visual Studio 10-15 "//&message=" + System.Net.WebUtility.UrlEncode(MessageText);// Visual Studio 12 try { WebRequest req = WebRequest.Create(URI); WebResponse resp = req.GetResponse(); var sr = new System.IO.StreamReader(resp.GetResponseStream()); return sr.ReadToEnd().Trim(); } catch (WebException ex) { var httpWebResponse = ex.Response as HttpWebResponse; if (httpWebResponse != null) { switch (httpWebResponse.StatusCode) { case HttpStatusCode.NotFound: return "404:URL not found :" + URI; break; case HttpStatusCode.BadRequest: return "400:Bad Request"; break; default: return httpWebResponse.StatusCode.ToString(); } } } return null; } }
API Parameters
username | Your Login Username (Required) |
---|---|
password | Your Login Password (Required) |
sender | Your company Name/Brand Name like "xyz" Displayed of Recipient Mobile (Required) |
mobile | Recipient Mobile Number (Required) |
message | Message For Recipient (Required) |
type | Type = unicode for unicode SMS (OPTIONAL) |
date | dd-mm-YYYY Example: 25-02-2014 (OPTIONAL) |
time | hh:mm:ss Example: 21:30:55 (OPTIONAL) |
format | Type is xml and json. Not Case sensitive. If set, the result will be returned according to the type specified. (OPTIONAL) |
Return Response and their Meanings
OK | The message was successfully accepted for delivery. |
---|---|
1 | Invalid Username Or Password |
2 | Please Type Username |
3 | Please Type Password |
4 | Please Type Sender ID |
-4 | Invalid Sender ID This Sender ID Is Not Register From P.T.A OR Sender ID Up to 11 Words Please Type Short Sender ID |
5 | Please Type Receiver Mobile Number |
6 | Please Type Your Message |
-6 | Message Length Exceeded More Than 612 Character (type = Text) OR Message Length Exceeded More Than 338 Character (type = unicode) |
8 | Low Credit Please Recharge Your Credit |
9 | SMS Rejected |
7 | Invalid Recipient Mobile Numbers Please Type Correct Mobile Number |
10 | Error! SMS Sending Failed Please Try Again Or Contact To Support |
11 | Invalid Date For Scheduled! |
Dynamic SMS HTTP API
bsms2.etsol.pk/web_distributor/api/personalized.php?username=&password=***&sender=BrandName&url=bsms2.etsol.pk/dynamic_sample.csv&message=Dear Parents!Your Son %23B%23 Got %23C%23 Marks In %23D%23, Your Son Result Is %23E%23 Please Contact With School For More Details
<?php $username = "";///Your Username $password = "password";///Your Password $sender = "SenderID"; $url = "bsms2.etsol.pk/dynamic_sample.csv"; $message = "Dear Parents! Your Son #B# Got #C# Marks In #D#, Your Son Result Is #E# Please Contact With School For More Details."; ////sending sms $post = "sender=".urlencode($sender)."&url=".$url."&message=".urlencode($message).""; $url = "bsms2.etsol.pk/web_distributor/api/personalized.php?username=&password=***"; $ch = curl_init(); $timeout = 30; // set to zero for no timeout curl_setopt($ch, CURLOPT_USERAGENT, 'Mozilla/4.0 (compatible; MSIE 6.0; Windows NT 5.1; SV1)'); curl_setopt($ch, CURLOPT_URL,$url); curl_setopt($ch, CURLOPT_POST, 1); curl_setopt($ch, CURLOPT_POSTFIELDS,$post); curl_setopt($ch, CURLOPT_RETURNTRANSFER, true); curl_setopt ($ch, CURLOPT_CONNECTTIMEOUT, $timeout); $result = curl_exec($ch); /*Print Responce*/ echo $result; ?>
Parameter For Dynamic SMS API
username | Your Login Username (Required) |
---|---|
password | Your Login Password (Required) |
sender | Your company Name/Brand Name like "xyz" Displayed of Recipient Mobile (Required) |
url | txt,csv,xls,xlsx File Link (Required) |
message | Message For Recipient (Required) |
duplicate | Type 0 For Remove Duplicate Numbers OR Type 1 For Don't Remove Duplicate Numbers (OPTIONAL) |
type | Type = unicode for unicode SMS (OPTIONAL) |
format | Type is xml and json. Not Case sensitive. If set, the result will be returned according to the type specified. (OPTIONAL) |
Return Response and their Meanings
OK | The message was successfully accepted for delivery. |
---|---|
1 | Invalid Username Or Password |
2 | Please Type Username |
3 | Please Type Password |
4 | Please Type Sender ID |
-4 | Invalid Sender ID This Sender ID Is Not Register From P.T.A OR Sender ID Up to 11 Words Please Type Short Sender ID |
5 | Please Type Receiver Mobile Number |
6 | Please Type Your Message |
-6 | Message Length Exceeded More Than 612 Character (type = Text) OR Message Length Exceeded More Than 338 Character (type = unicode) |
8 | Low Credit Please Recharge Your Credit |
9 | SMS Rejected |
7 | Invalid Recipient Mobile Numbers Please Type Correct Mobile Number |
10 | Error! SMS Sending Failed Please Try Again Or Contact To Support |
11 | Invalid Date For Scheduled! |
Note: Download csv sample file
HTTP API For Delivery Reports
bsms2.etsol.pk/web_distributor/api/delivery.php?username=&password=***&id=***
<?php $username = "";///Your Username $password = "password";///Your Password $id = '***';////unique message id ////Getting Delivery Reports $post = "id=".$id." "; $url = "bsms2.etsol.pk/web_distributor/api/delivery.php?username=".$username."&password=".$password.""; $ch = curl_init(); $timeout = 30; // set to zero for no timeout curl_setopt($ch, CURLOPT_USERAGENT, 'Mozilla/4.0 (compatible; MSIE 6.0; Windows NT 5.1; SV1)'); curl_setopt($ch, CURLOPT_URL,$url); curl_setopt($ch, CURLOPT_POST, 1); curl_setopt($ch, CURLOPT_POSTFIELDS,$post); curl_setopt($ch, CURLOPT_RETURNTRANSFER, true); curl_setopt ($ch, CURLOPT_CONNECTTIMEOUT, $timeout); $result = curl_exec($ch); /*Print Responce*/ echo $result; ?>
API Parameters
username | Your Login Username (Required) |
---|---|
password | Your Login Password (Required) |
id | Unique Message ID (Required) |
Delivery Report Documentation
Examples
Each Message Returns A Unique Identifier in The Form Of Transaction ID.
URL To Get Single Message Delivery
http:/sms.futuresol.net/web_distributor/api/delivery.php?username=&password=***&id=a2371b64-4da5-44d5-9955-34c82682c8d0
JSON Success Response
{"success":"true","results":[{"id":"260","api_id":"a2371b64-4da5-44d5-9955-34c82682c8d0","delivery":"Sent","sender":"SMS Alert","mobile":"923101234567","length":"11","price":"1","operator":"Zong (Paktel)","country":"Pakistan","date":"15:41:06 PM 19-09-17"}]}
URL To Get Multiple Message Delivery
bsms2.etsol.pk/web_distributor/api/delivery.php?username=&password=***&id=a2371b64-4da5-44d5-9955-34c82682c8d0,89936c10-79ba-4252-84d5-e0c555405713
JSON Success Response
{"success":"true","results":[{"id":"260","api_id":"a2371b64-4da5-44d5-9955-34c82682c8d0","delivery":"Sent","sender":"DD Labs","mobile":"923001234567","length":"11","price":"1","operator":"Zong (Paktel)","country":"Pakistan","date":"15:41:06 PM 19-09-17"},{"id":"256","api_id":"89936c10-79ba-4252-84d5-e0c555405713","delivery":"Sent","sender":"DD Labs","mobile":"923101234567","length":"4","price":"1","operator":"Zong (Paktel)","country":"Pakistan","date":"12:46:23 PM 19-09-17"}]}
JSON ERROR Response
{"success":"false","results":[{"status":"5","error":"Invalid Message ID"}]}
Error Codes Descriptions
ERROR Codes
Status | Description |
---|---|
Success | false |
1 | Invalid Username Or Password |
2 | Please Type Username |
3 | Please Type Password |
4 | Please Type Delivery ID |
5 | Invalid Message ID |
Success Response Description
Status | Description |
---|---|
Success | true |
Id | Message Delivery Id i.e. (a2371b64-4da5-44d5-9955-34c82682c8d0) |
delivery | Delivery Status i.e. (Delivered , Failed , Sent ) |
sender | Masking ID i.e. (SMS Alert) |
mobile | Recipient Mobile Number i.e. (923001234567) |
length | Message Length i.e. (142 Characters) |
price | Submitted Message Price i.e. (0.02) |
operator | Network Operator i.e. (Mobilink) |
country | Country Name i.e. (Pakistan) |
date | Sent Message Time (17:45:10 PM 07-12-15) |
HTTP API For Balance
bsms2.etsol.pk/web_distributor/api/balance_check.php?username=&password=***
<?php $username = '';///Your Username $password = 'password';///Your Password $url = "bsms2.etsol.pk/web_distributor/api/balance_check.php?username=".$username."&password=".$password.""; $ch = curl_init(); $timeout = 30; curl_setopt ($ch,CURLOPT_URL, $url) ; curl_setopt ($ch,CURLOPT_RETURNTRANSFER, 1); curl_setopt ($ch,CURLOPT_CONNECTTIMEOUT, $timeout) ; $response = curl_exec($ch) ; curl_close($ch) ; //Write out the response echo $response ; ?>
API Parameters
username | Your Login Username (Required) |
---|---|
password | Your Login Password (Required) |
format | Type is xml and json. Not Case sensitive. If set, the result will be returned according to the type specified. (OPTIONAL) |
Return Response and their Meanings
OK | Successful Result. |
---|---|
1 | Invalid Username Or Password |
2 | Please Type Username |
3 | Please Type Password |
Our Clients
bsms2.etsol.pk team is fully supported service of bulk SMS with clients.We provide of SMS Service offering SMS Marketing, Voice SMS and Bulk SMS with the latest technology and Marketing Solutions. Our team is here to available for customer helping.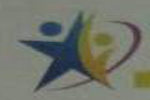
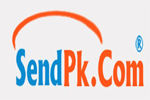
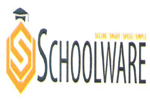
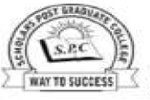
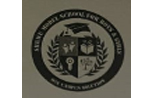
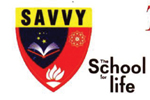
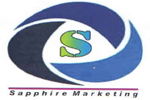
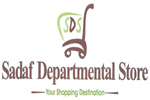
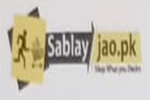
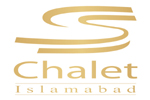
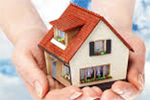
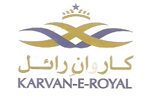
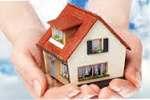
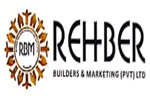
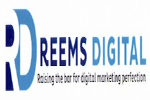
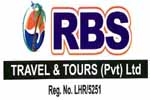
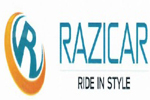